Evaluating Data with Python
When receiving data from the server, you are correct that using a for loop will result in a list error. However, there are several ways to evaluate and process the data more efficiently.
Here is an example of how to parse and return specific data from a JSON response in Python:
JSON Response Default
[
{
"id": 1,
"name": "John Doe",
"age": 30,
"city": "New York"
},
{
"id": 2,
"name": "Jane Smith",
"age": 25,
"city": "Los Angeles"
}
]
Using Python to parse and return data
import json
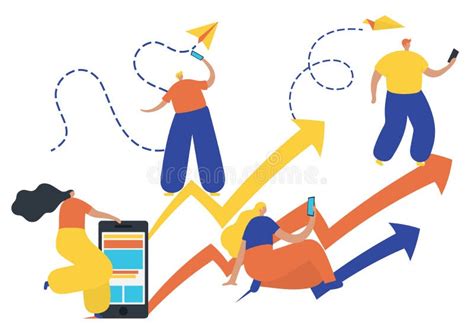
Assuming the JSON response is stored in a variable named "data"data = [
{"id": 1, "name": "John Doe", "age": 30, "city": "New York"},
{"id": 2, "name": "Jane Smith", "age": 25, "city": "Los Angeles"}
]
Define a function to extract given datadef get_data(data):
keys_to_extract = ["id", "name", "age"]
for an item in the data:
extracted_data = {}
for keys_to_extract:
if you enter an item:
extracted_data[key] = address[key]
yield extracted_data
Example usageperson get_data(data):
print (person)
In this example, we define a function “get_data” that takes a list of objects (“data”) and returns a generator expression that produces dictionaries containing specified data (e.g. “id”, “name”, and “age”). We then use the “usage example” section to demonstrate how to reproduce the extracted data.
Using certain information
You can simply repeat the generator expression to access specific data:
person get_data(data):
print(person["id"])
print: 1print(person["name"])
print: John Doe
Alternatively, if you want to use a for loop instead of a generator expression, you can define a separate function that takes the data as an argument:
def get_person(person):
return {"id": person["id"], "name": person["name"]}
Example usagedata = [
{"id": 1, "name": "John Doe", "age": 30, "city": "New York"},
{"id": 2, "name": "Jane Smith", "age": 25, "city": "Los Angeles"}
]
for the person in the data:
print(get_person(person))
This approach is better suited when working with larger data sets that do not fit in memory.